TypeScript Essentials |
Course Outline |
Outline
|
Reading Material |
Download
|
Software |
|
Setup Guide |
Setup Guide.pdf
|
Day | Modules | Sharing |
1 |
- What is TypeScript?
- Features of TypeScript
- Why Use TypeScript?
- Components of TypeScript
- Local Environment Setup
- Installing Node.js
- IDE Support
- Visual Studio Code
- Your First TypeScript Code
- Compile and Execute a TypeScript Program
- Compiler Flags
- Identifiers in TypeScript
- TypeScript Keywords
- Comments in TypeScript
- TypeScript and Object Orientation
- The Any type
- Built-in types
- User-defined Types
- Variable Declaration in TypeScript
- Type Assertion in TypeScript
- Inferred Typing in TypeScript
- TypeScript Variable Scope
- What is an Operator?
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Miscellaneous Operators
- Type Operators
- If-Then
- If-Then-Else
- Else-If
- Switch statement
- Definite Loop
- Indefinite Loop
- The break Statement
- The continue Statement
- The Infinite Loop
- Optional Parameters
- Rest Parameters
- Default Parameters
- Anonymous Function
- The Function Constructor
- Recursion and TypeScript Functions
- Lambda Functions
- Syntactic Variations
- Function Overloads
|
|
2 |
- Features of an Array
- Declaring and Initializing Arrays
- Accessing Array Elements
- Array Object
- Array Methods
- Array Destructuring
- Array Traversal using for…in loop
- Arrays in TypeScript
- Syntax
- Accessing values in Tuples
- Tuple Operations
- Updating Tuples
- Destructuring a Tuple
- Syntax
- Union Type and Arrays
- Declaring Interfaces
- Union Type and Interface
- Interfaces and Arrays
- Interfaces and Inheritance
- Creating classes
- Creating Instance objects
- Accessing Attributes and Functions
- Class Inheritance
- Class inheritance and Method Overriding
- The static Keyword
- The instanceof operator
- Data Hiding
- Classes and Interfaces
- Syntax
- TypeScript Type Template
- Duck-typing
- Defining a Namespace
- Nested Namespaces
- Internal Module
- External Module
- Purpose
- Defining Ambients
|
|
3 |
Mini-Project
|
|
Recommanded Books |
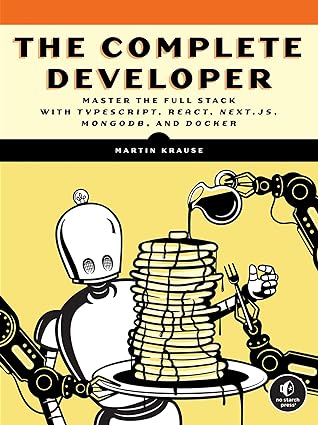 | Title | The Complete Developer: Master the Full Stack with TypeScript, React, Next.js, MongoDB, and Docker |
ISBN | 978-1-718-50328-1 |
Author | Martin Krause |
Year | 2024 |
Publisher | No Starch Press |
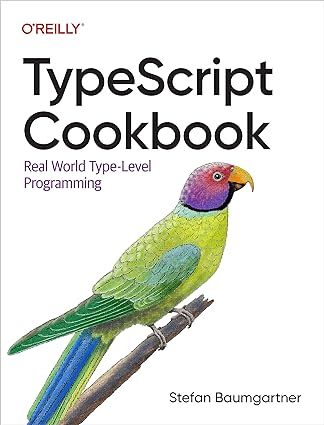 | Title | TypeScript Cookbook: Real World Type-Level Programming |
ISBN | 978-1-098-13665-9 |
Author | Stefan Baumgartner |
Year | 2023 |
Publisher | O'Reilly Media, Inc. |
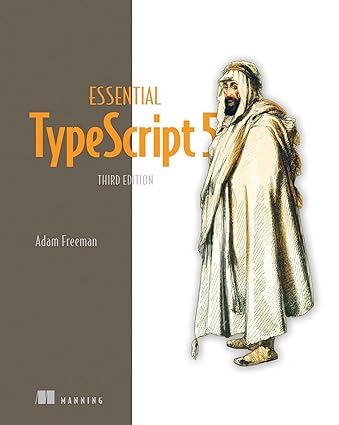 | Title | Essential TypeScript 5, Third Edition (MEAP V02). |
ISBN | 978-1-633-43731-9 |
Author | Adam Freeman |
Year | 2023 |
Publisher | Manning Publications Co. |
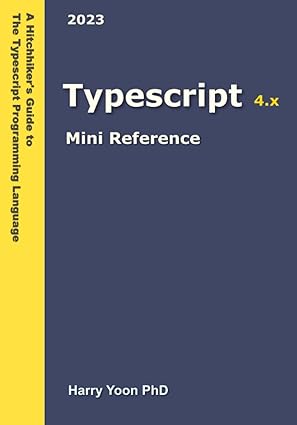 | Title | Typescript Mini Reference: A Quick Guide to the Typescript Programming Language for Busy Coders |
ISBN | B0BSZVKW5R |
Author | Harry Yoon |
Year | 2023 |
Publisher | Coding Books Press |
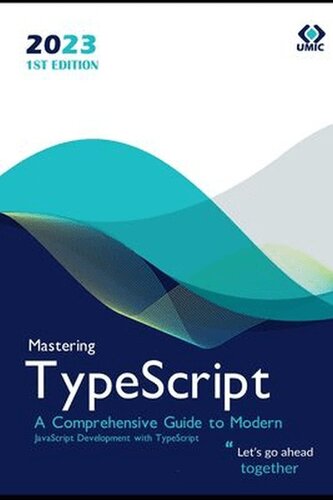 | Title | Mastering TypeScript: A Comprehensive Guide to Modern JavaScript Development with TypeScript |
ISBN | 979-8-223-67396-5 |
Author | Eliza Rosewood |
Year | 2023 |
Publisher | Ali Alakbar Mohammed Radhi |
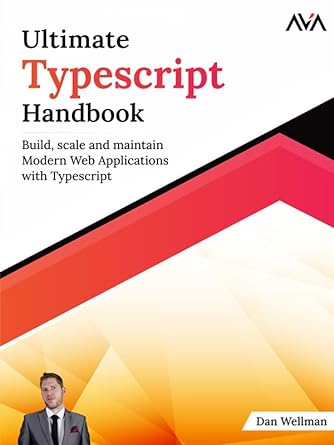 | Title | Ultimate Typescript Handbook: Build, scale and maintain Modern Web Applications with Typescript |
ISBN | 978-9-388-59078-5 |
Author | Dan Wellman |
Year | 2023 |
Publisher | Orange Education PVT Ltd |
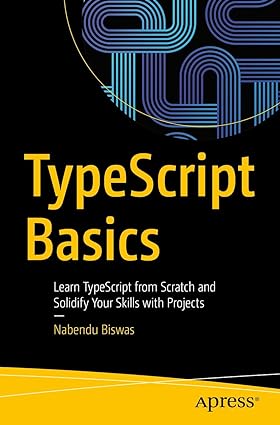 | Title | TypeScript Basics: Learn TypeScript from Scratch and Solidify Your Skills with Projects |
ISBN | 978-1-484-29522-9 |
Author | Nabendu Biswas |
Year | 2023 |
Publisher | Apress |
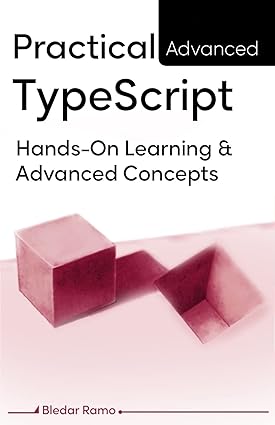 | Title | Practical Advanced TypeScript: Hands-On Learning And Advanced Concepts |
ISBN | B0C4QXBW74 |
Author | Bledar Ramo |
Year | 2023 |
Publisher | Independently published |
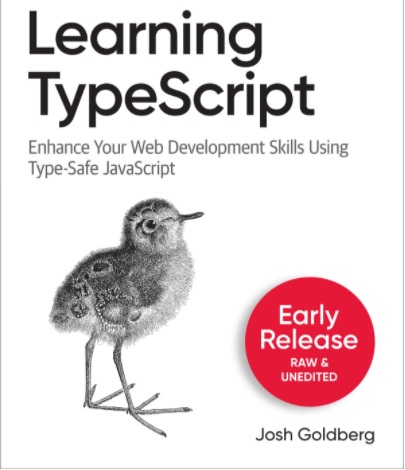 | Title | Learning TypeScript |
ISBN | 978-1-098-11031-4 |
Author | Josh Goldberg |
Year | 2022 |
Publisher | O'Reilly Media, Inc. |
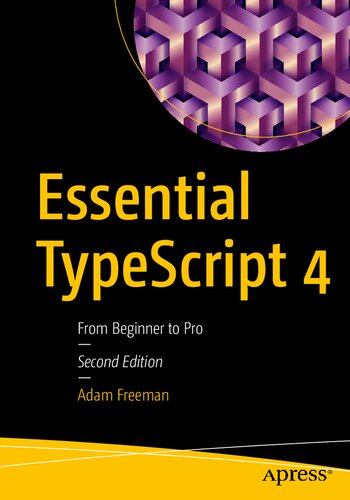 | Title | Essential TypeScript 4: From Beginner to Pro |
ISBN | 978-1-484-27010-3 |
Author | Adam Freeman |
Year | 2021 |
Publisher | Apress |
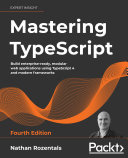 | Title | Mastering TypeScript: Build enterprise-ready, modular web applications using TypeScript 4 and modern frameworks, 4th Edition |
ISBN | 978-1-800-56160-1 |
Author | Nathan Rozentals |
Year | 2021 |
Publisher | Packt Publishing Ltd |
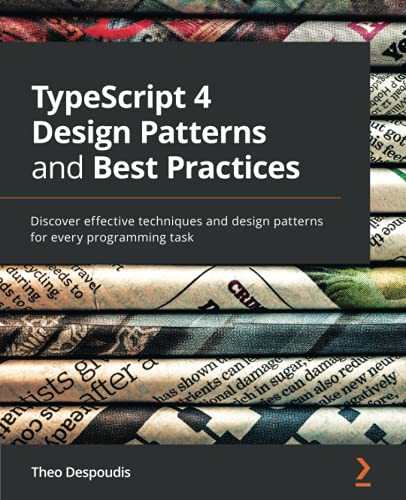 | Title | TypeScript 4 Design Patterns and Best Practices: Discover effective techniques and design patterns for every programming task |
ISBN | 978-1-800-56342-1 |
Author | Theo Despoudis |
Year | 2021 |
Publisher | Packt Publishing |
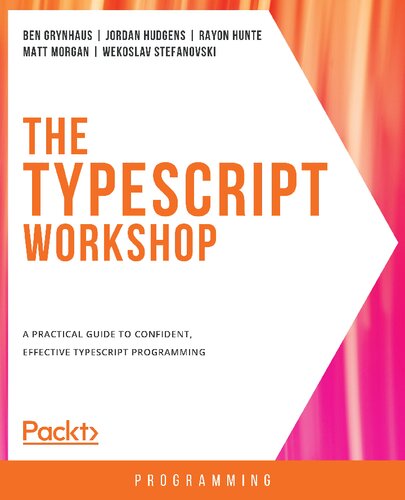 | Title | The TypeScript Workshop: A practical guide to confident, effective TypeScript programming |
ISBN | 978-1-838-82849-3 |
Author | Ben Grynhaus, Jordan Hudgens, Rayon Hunte, Matthew Thomas Morgan, Wekoslav Stefanovski |
Year | 2021 |
Publisher | Packt Publishing |
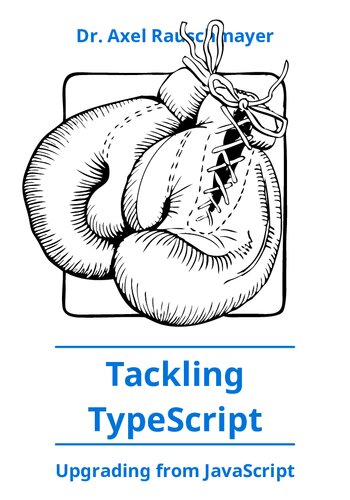 | Title | Tackling TypeScript |
ISBN | |
Author | Rauschmayer, Axel |
Year | 2020 |
Publisher | |
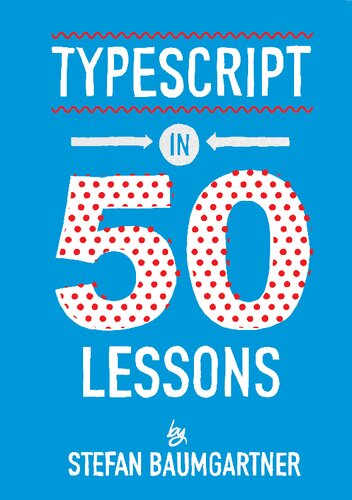 | Title | TypeScript in 50 Lessons |
ISBN | 978-3-945-74990-6 |
Author | Stefan Baumgartner |
Year | 2020 |
Publisher | Smashing Magazine |
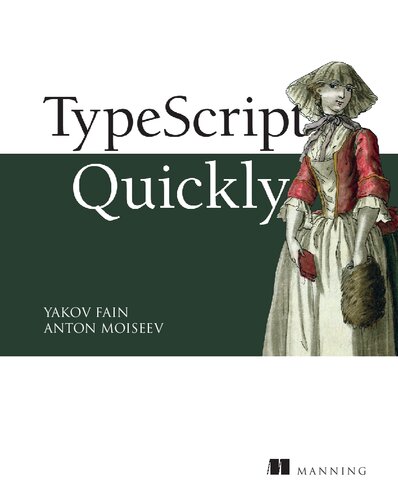 | Title | TypeScript Quickly |
ISBN | 978-1-617-29594-2 |
Author | Yakov Fain, Anton Moisee |
Year | 2020 |
Publisher | Manning Publications |
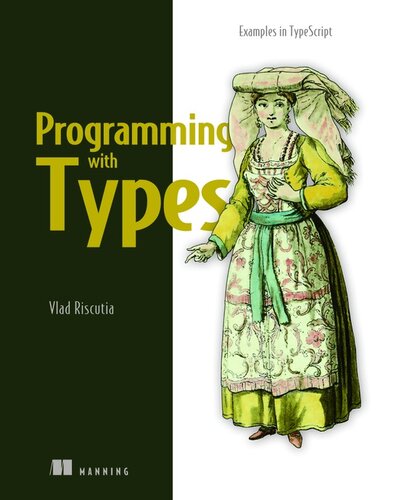 | Title | Programming with Types: Examples in TypeScript |
ISBN | 978-1-617-29641-3 |
Author | Vlad Riscutia |
Year | 2020 |
Publisher | Manning Publications |
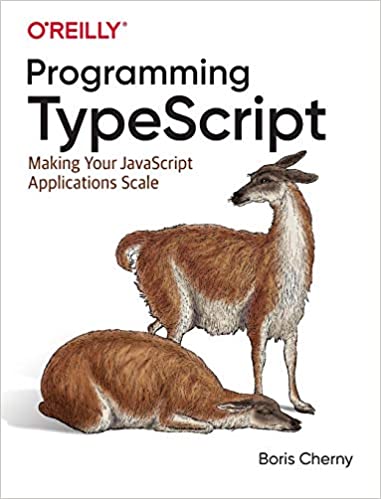 | Title | Programming TypeScript |
ISBN | 978-1-492-03765-1 |
Author | Boris Cherny |
Year | 2019 |
Publisher | O’Reilly |
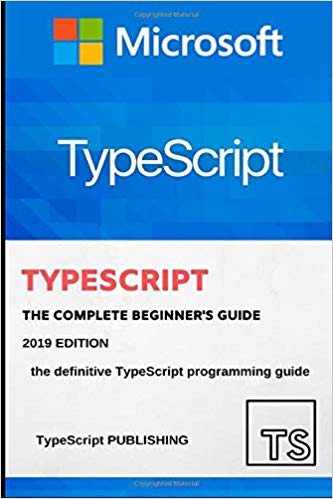 | Title | TypeScript Programming Language |
ISBN | 978-1-708-83980-2 |
Author | TypeScript Publishing |
Year | 2019 |
Publisher | TypeScript Publishing |
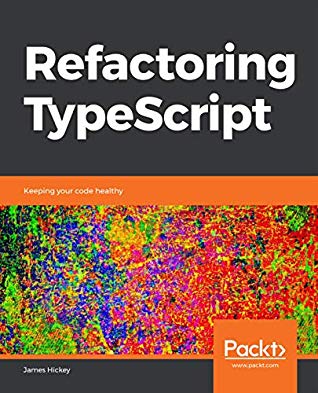 | Title | Refactoring TypeScript: Keeping your code healthy |
ISBN | 978-1-839-21804-0 |
Author | James Hickey |
Year | 2019 |
Publisher | Packt Publishing |
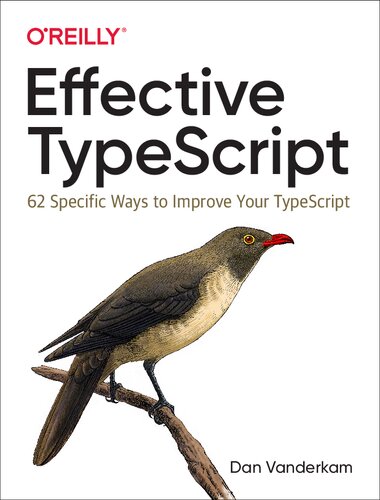 | Title | Effective TypeScript: 62 Specific Ways to Improve Your TypeScript |
ISBN | 978-1-492-05371-2 |
Author | Dan Vanderkam |
Year | 2019 |
Publisher | O'Reilly Media |
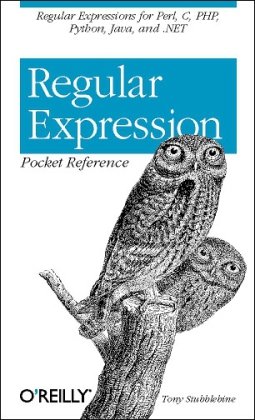 | Title | Regular Expression Pocket Reference, 2nd Edition |
ISBN | 978-0-596-51427-3 |
Author | Tony Stubblebine |
Year | 2007 |
Publisher | O'Reilly Media |
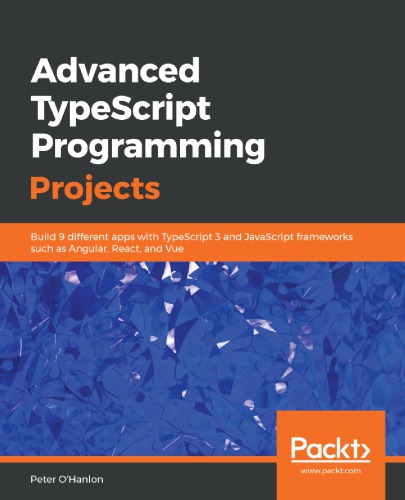 | Title | Advanced TypeScript Programming Projects |
ISBN | 978-1-789-13304-2 |
Author | O'Hanlon, Peter |
Year | 2019 |
Publisher | Packt Publishing |